- Error: Unable to locate Android SDK
- Error: cmdline-tools component is missing
- Error: An InputDecorator, which is typically created by a TextField, cannot have an unbounded width
- Error: Couldn’t resolve the package ‘path_provider’
- Error: Couldn’t resolve the package ‘sqflite’
- Error: I cannot find the java executable
Error: Unable to locate Android SDK
Problem
After executing flutter doctor the following errors are issued:
>flutter doctor
Doctor summary (to see all details, run flutter doctor -v):
[√] Flutter (Channel stable, 3.0.5, on Microsoft Windows [Version 10.0.22000.795], locale en-CH)
[X] Android toolchain - develop for Android devices
X Unable to locate Android SDK.
Install Android Studio from: https://developer.android.com/studio/index.html
On first launch it will assist you in installing the Android SDK components.
(or visit https://flutter.dev/docs/get-started/install/windows#android-setup for detailed instructions).
If the Android SDK has been installed to a custom location, please use
`flutter config --android-sdk` to update to that location.
[√] Chrome - develop for the web
[!] Visual Studio - develop for Windows (Visual Studio Community 2022 17.2.3)
X Visual Studio is missing necessary components. Please re-run the Visual Studio installer for the "Desktop
development with C++" workload, and include these components:
MSVC v142 - VS 2019 C++ x64/x86 build tools
- If there are multiple build tool versions available, install the latest
C++ CMake tools for Windows
Windows 10 SDK
[√] Android Studio (version 2021.2)
[√] VS Code (version 1.69.2)
[√] Connected device (3 available)
[√] HTTP Host Availability
! Doctor found issues in 2 categories.
Resolution
For Windows: Inside Android Studio, get path from: gear icon > Apprearance & Behavior > System Settings > Android SDK > Android SDK Location


Make sure that the location path does not contain any spaces in it. In case the location path has any spaces, remove them from the path and re-install the SDK to the new corrected location.
After that execute the following commands:
flutter config --android-sdk {path}
flutter doctor --android-licenses
Error: cmdline-tools component is missing
Problem
After executing flutter doctor the following errors are issued:
>flutter doctor
Doctor summary (to see all details, run flutter doctor -v):
[√] Flutter (Channel stable, 3.0.5, on Microsoft Windows [Version 10.0.22000.795], locale en-CH)
[!] Android toolchain - develop for Android devices (Android SDK version 33.0.0)
X cmdline-tools component is missing
Run `path/to/sdkmanager --install "cmdline-tools;latest"`
See https://developer.android.com/studio/command-line for more details.
X Android license status unknown.
Run `flutter doctor --android-licenses` to accept the SDK licenses.
See https://flutter.dev/docs/get-started/install/windows#android-setup for more details.
[√] Chrome - develop for the web
[!] Visual Studio - develop for Windows (Visual Studio Community 2022 17.2.3)
X Visual Studio is missing necessary components. Please re-run the Visual Studio installer for the "Desktop
development with C++" workload, and include these components:
MSVC v142 - VS 2019 C++ x64/x86 build tools
- If there are multiple build tool versions available, install the latest
C++ CMake tools for Windows
Windows 10 SDK
[√] Android Studio (version 2021.2)
[√] VS Code (version 1.69.2)
[√] Connected device (3 available)
[√] HTTP Host Availability
! Doctor found issues in 2 categories.
Potential Resolution
Install Android SDK Command-line Tools:
Preferences
> Appearance & Behavior
> System Settings
> Android SDK
> SDK Tools
>

Check this option:
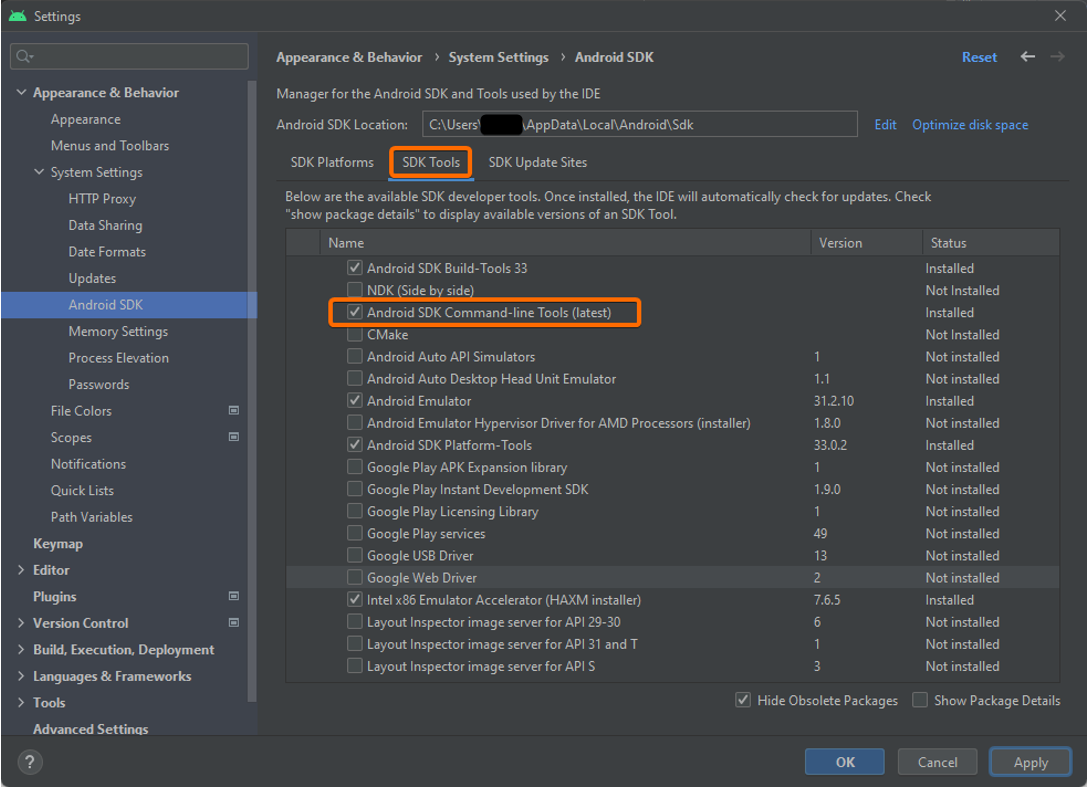
Accept Android Licences by executing the following command:
flutter doctor --android-licenses
Error: An InputDecorator, which is typically created by a TextField, cannot have an unbounded width
Problem
I have the following code:
import 'package:flutter/material.dart';
void main() => runApp(const MaterialApp(
home: SampleApp()
));
class SampleApp extends StatelessWidget {
const SampleApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Sample Application'),
),
body: Center(
child: Row(
children: const [
Text('Family Name:'),
TextField(
decoration: InputDecoration(
hintText: 'Enter a value',
),
),
],
),
),
);
}
}
Trying to compile that code leads to the following error being issued:
An InputDecorator, which is typically created by a TextField, cannot have an unbounded width.
This happens when the parent widget does not provide a finite width constraint. For example, if the InputDecorator is contained by a Row, then its width must be constrained. An Expanded widget or a SizedBox can be used to constrain the width of the InputDecorator or the TextField that contains it.
Resolution
To resolve this issue change the code as follows:
import 'package:flutter/material.dart';
void main() => runApp(const MaterialApp(
home: SampleApp()
));
class SampleApp extends StatelessWidget {
const SampleApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Sample Application'),
),
body: Center(
child: Row(
children: const [
Text('Family Name:'),
Expanded(
child: TextField(
decoration: InputDecoration(
hintText: 'Enter a value',
),
),
)
],
),
),
);
}
}
Error: Couldn’t resolve the package ‘path_provider’
Problem
We try to import the following package:
import 'package:path_provider/path_provider.dart';
And the compiler issues the following error:
Performing hot reload...
Syncing files to device sdk gphone64 x86 64...
Error: Couldn't resolve the package 'path_provider' in 'package:path_provider/path_provider.dart'.
lib/main.dart:3:8: Error: Not found: 'package:path_provider/path_provider.dart'
import 'package:path_provider/path_provider.dart';
^
Resolution
Add the package path_provider to the pubspec.yaml
file:
pubspec.yaml
:
...
# dependencies can be manually updated by changing the version numbers below to
# the latest version available on pub.dev. To see which dependencies have newer
# versions available, run `flutter pub outdated`.
dependencies:
flutter:
sdk: flutter
shared_preferences: "2.0.15"
path_provider: ^2.0.0
...
Error: Couldn’t resolve the package ‘sqflite’
Problem
We try to import the following package:
import "package:sqflite/sqflite.dart";
And the compiler issues the following error:
Performing hot reload...
Syncing files to device sdk gphone64 x86 64...
Error: Couldn't resolve the package 'sqflite' in 'package:sqflite/sqflite.dart'.
lib/main.dart:2:8: Error: Not found: 'package:sqflite/sqflite.dart'
import 'package:sqflite/sqflite.dart';
^
Resolution
Add the package sqflite to the pubspec.yaml
file:
pubspec.yaml
:
...
# dependencies can be manually updated by changing the version numbers below to
# the latest version available on pub.dev. To see which dependencies have newer
# versions available, run `flutter pub outdated`.
dependencies:
flutter:
sdk: flutter
sqflite:
...
Error: I cannot find the java executable
Problem
Trying to run a dart project results in the following error being issued:
Launching lib\main.dart on sdk gphone64 x86 64 in debug mode...
Running Gradle task 'assembleDebug'...
FAILURE: Build failed with an exception.
* What went wrong:
The supplied javaHome seems to be invalid. I cannot find the java executable. Tried location: C:\Program Files\Android\Android Studio\jre\bin\java.exe
* Try:
> Run with --stacktrace option to get the stack trace.
> Run with --info or --debug option to get more log output.
> Run with --scan to get full insights.
* Get more help at https://help.gradle.org
Exception: Gradle task assembleDebug failed with exit code 1
Debugging
You might run the application from the command line instead of from Android Studio GUI to better debug this problem. You can do this by navigating to the root of your dart project and running the following command:
flutter run -v
Potential Resolution
Idea from this discussion: https://discuss.gradle.org/t/gradle-is-trying-to-use-a-jdk-that-has-been-uninstalled-from-my-system/43202/5
In the case of a Windows system navigate to the following path:
C:\Users\UserName\.gradle\daemon\7.4
First backup, then delete the following file:
registry.bin
Then re-run your dart application, either from Android Studio via GUI or by the following command:
flutter run